Astro: Add JSON-LD Structured Data to Your Website for Rich Search Results
When building a modern website, structured data can enhance SEO and aid search engines with understanding your content. This guide walks you through creating a reusable component to dynamically add JSON-LD structured data tailored to each rendered page on your Astro website.
Instructions
Step 1: New Component to Render Structured Data
Begin by creating a new component called HeadMeta.astro
. This component adds globally shared elements in the HTML <head>
section, such as <title>
, <meta>
, <link>
, <style>
, and <script>
. We’ll also use it to include structured data as a <script>
element with the type
set to application/ld+json
.
Below is an excerpt from the HeadMeta.astro
component used on this website. The structured data follows the schemas defined at Schema.org.
In the component script section (code fence), the structuredData
variable stores the necessary types and properties for the rendered structured data. These properties may vary depending on the type of page. For example, every page will include structured data defining the WebSite
type, but pages may also include BlogPosting
or Article
types, depending on the content.
For the WebSite
schema, we define the website name, description, homepage URL, and some information about the publisher (e.g., my name, a link to my homepage, and links to external profiles like LinkedIn or social media profiles). I’ve used “Aida Bugg” as an example, but replace that with your own name unless that happens to be yours.
Additionally, we define a new Astro.prop
called type
. As we’ll see later in the guide, this property determines whether the page uses the BlogPosting
or Article
type.
---
import { SITE_TITLE, SITE_DESCRIPTION } from '../consts';
const canonicalURL = new URL(Astro.url.pathname, Astro.url);
const siteURL = new URL("/", Astro.url);
const webSiteSchema = new URL("/#/schema/WebSite", Astro.url);
const personSchema = new URL("/#/schema/Person/aidabugg", Astro.url);
const { type, title, description, pubDate, updatedDate } = Astro.props;
const structuredData = {
"@context": "https://schema.org",
"@graph": [
{
"@type": "WebSite",
"@id": webSiteSchema,
name: SITE_TITLE,
description: SITE_DESCRIPTION,
url: siteURL,
publisher: {
"@type": "Person",
"@id": personSchema,
name: "Aida Bugg",
url: siteURL,
sameAs: [
"https://www.example.com/profile1/aida_bugg/",
"https://www.example.com/profile2/aida_bugg/"
]
}
},
...type ?
[{
"@type": type,
"@id": canonicalURL,
url: canonicalURL,
name: title,
headline: title,
description: description,
isPartOf: {
"@id": webSiteSchema,
},
datePublished: pubDate,
dateModified: updatedDate,
author: {
"@type": "Person",
"@id": personSchema,
}
}] : []
]
}
---
Add the following line to the component template section. This will render the structured data into the HTML of any Astro page where the HeadMeta.astro
component is imported and used.
<script type="application/ld+json" set:html={JSON.stringify(structuredData)} />
Step 2: Blog Posts
For blog posts, I use a common layout defined in StandardPostLayout.astro
. First, add an import statement for the HeadMeta.astro
component to the code fence. No changes are needed for Astro.props
.
---
import HeadMeta from '../components/HeadMeta.astro';
const { title, description, pubDate, updatedDate } = Astro.props;
---
Next, modify the post shell to include the HeadMeta
component and pass the necessary properties. The type
property is hardcoded as BlogPosting
. For pages using the StandardPostLayout.astro
layout, the structured data will include both the WebSite
and BlogPosting
types with their respective properties.
<head>
<HeadMeta
type="BlogPosting"
title={title}
description={description}
pubDate={pubDate}
updatedDate={updatedDate}
/>
</head>
Step 3: Pages
For pages, I use a common layout defined in StandardPageLayout.astro
. Again, modify the layout code fence to import the HeadMeta.astro
component. No changes are needed for Astro.props
.
---
import HeadMeta from '../components/HeadMeta.astro';
const { type, title, description } = Astro.props;
---
Modify the page shell to include the HeadMeta
component and pass the appropriate properties. In this case, the type
property is not hardcoded, allowing it to be passed from the individual page.
<head>
<HeadMeta
type={type}
title={title}
description={description}
/>
</head>
In the individual page files that use the StandardPageLayout.astro
layout, you can now set the type
to Article
.
<Layout type="Article" title="Page Title" description="Page Description"></Layout>
On my website, I only use the Article
type for pages, but this approach lets you pass other schema types as needed. The structured data will include both the WebSite
and Article
types with their respective properties.
Results
The structured data is correctly rendered in the <head>
element of each page. Inspecting the HTML shows that a blog post includes both the WebSite
and BlogPosting
types, while a page includes both the WebSite
and Article
types. Below are screenshots from my website as examples.
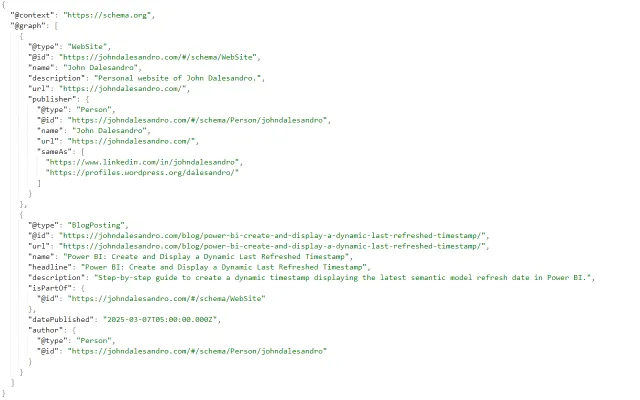
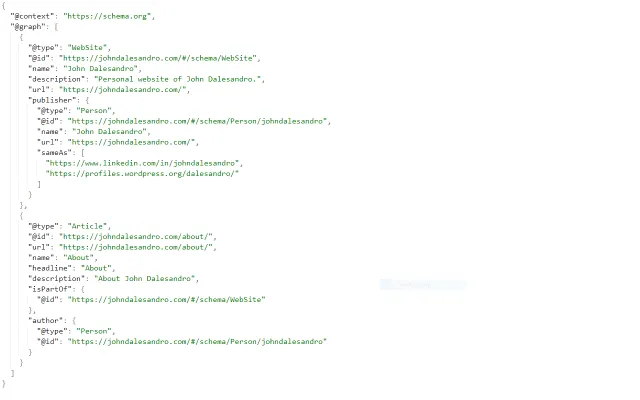
Summary
By integrating structured data, you ensure your Astro website effectively communicates content types to search engines, potentially boosting SEO and enhancing discoverability. This flexible approach allows you to include WebSite, BlogPosting, and Article schemas tailored to each rendered page. With structured data in place, your website may now be better positioned for richer search results and improved online visibility.