Excel VBA: Retrieving Outlook Address Book Values
The following custom Excel VBA function queries the Outlook address book for a specific name or alias and returns the requested attribute or property value. The function supports both local contacts as well as Exchange address books.
Instructions
Enable Microsoft Outlook Object Library
To use this function, you must enable the Microsoft Outlook 16.0 Object Library
. The version number varies based on the installed version of Microsoft Excel.
- From the
Developer
ribbon in Microsoft Excel, clickVisual Basic
. - Once the
Visual Basic for Applications
window opens, go to theTools
menu and selectReferences…
. - From the
References
dialog box, check/enableMicrosoft Outlook 16.0 Object Library
. - Click the
OK
button.
Create a New VBA Module
A new module is needed to store the function code if one does not already exist in the workbook. If the code is added directly to a worksheet object, then it will not work properly.
- From the
Developer
ribbon in Excel, clickVisual Basic
. - In the
Visual Basic for Applications
window, go toInsert
>Module
. - Paste the following code into the new module and close the editor.
Option Explicit
Public Function GetOutlookAddressBookProperty( _
ByVal alias As String, _
ByVal propertyName As String _
) As Variant
On Error GoTo ErrorHandler
Dim olApp As Outlook.Application
Dim olNameSpace As Namespace
Dim olRecipient As Outlook.Recipient
Dim olExchUser As Outlook.ExchangeUser
Dim olContact As Outlook.AddressEntry
Set olApp = CreateObject("Outlook.Application")
Set olNameSpace = olApp.GetNamespace("MAPI")
Set olRecipient = olNameSpace.CreateRecipient(LCase(Trim(alias)))
olRecipient.Resolve
If olRecipient.Resolved Then
Set olExchUser = olRecipient.AddressEntry.GetExchangeUser
If Not olExchUser Is Nothing Then
GetOutlookAddressBookProperty = Switch( _
propertyName = "Job Title", olExchUser.JobTitle, _
propertyName = "Company Name", olExchUser.CompanyName, _
propertyName = "Department", olExchUser.Department, _
propertyName = "Name", olExchUser.Name, _
propertyName = "First Name", olExchUser.FirstName, _
propertyName = "Last Name", olExchUser.LastName, _
propertyName = "Country/Region", _
olExchUser.PropertyAccessor.GetProperty( _
"http://schemas.microsoft.com/mapi/proptag/0x3A26001E") _
)
Else
Set olContact = olRecipient.AddressEntry
If Not olContact Is Nothing Then
With olContact.GetContact
GetOutlookAddressBookProperty = Switch( _
propertyName = "Job Title", .JobTitle, _
propertyName = "Company Name", .CompanyName, _
propertyName = "Department", .Department, _
propertyName = "Name", .FullName, _
propertyName = "First Name", .FirstName, _
propertyName = "Last Name", .LastName, _
propertyName = "Country/Region", _
.BusinessAddressCountry _
)
End With
Else
GetOutlookAddressBookProperty = CVErr(xlErrNA)
End If
End If
Else
GetOutlookAddressBookProperty = CVErr(xlErrNA)
End If
Exit Function
ErrorHandler:
If Err.Number <> 0 Then
GetOutlookAddressBookProperty = CVErr(xlErrNA)
End If
End Function
Usage
The GetOutlookAddressBookProperty
function is now usable in workbook formulas.
=GetOutlookAddressBookProperty(alias, propertyName)
The alias
parameter accepts any string value, but the function works best if the value is a uniquely resolvable attribute of an address book entry, e.g., full name, alias name, alias, etc. The function fails if it is unable to resolve to an individual entry in the address book.
The propertyName
parameter accepts the following values:
- Job Title
- Company Name
- Department
- Name
- First Name
- Last Name
- Country/Region
Results
Here’s an example Outlook address book entry used to demonstrate the function.
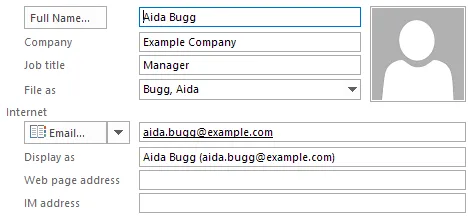
The GetOutlookAddressBookProperty
function retrieves various property values from Outlook using an Excel formula.
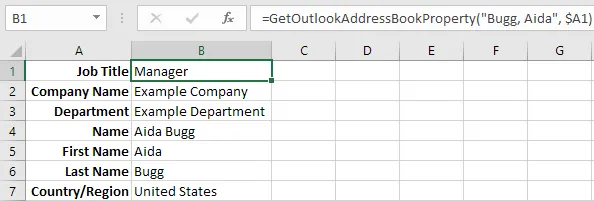
Further Enhancements
While this example only exposes a few properties, many others are available in the ExchangeUser Interface.
You may notice that accessing Country/Region
is slightly different because it uses the PropertyAccessor
to retrieve the value for an Exchange user. For reference, Microsoft has a list of MAPI Property Tags. However, it is no longer maintained so please be aware that it may be outdated. As an example, the Unicode property tag value for Country/Region
is not listed.
There are actively maintained tools available to help identify MAPI property tags, e.g., MFCMAPI and Outlook Spy (which also publishes a list of MAPI Property Tags). In this code, the Country/Region
field corresponds to the PR_BUSINESS_ADDRESS_COUNTRY
property tag which has a property tag value of 0x3A26001F
.
Similarly, phone numbers are accessed differently depending on whether it is a local contact or Exchange user.
For local contact phone numbers, the following properties are available (among others):
olContact.GetContact.BusinessTelephoneNumber
olContact.GetContact.HomeTelephoneNumber
olContact.GetContact.MobileTelephoneNumber
For Exchange entries, the following properties are available for PR_BUSINESS_TELEPHONE_NUMBER
, PR_HOME_TELEPHONE_NUMBER
, and PR_MOBILE_TELEPHONE_NUMBER
respectively.
olExchUser.PropertyAccessor.GetProperty("http://schemas.microsoft.com/mapi/proptag/0x3A08001F")
olExchUser.PropertyAccessor.GetProperty("http://schemas.microsoft.com/mapi/proptag/0x3A09001F")
olExchUser.PropertyAccessor.GetProperty("http://schemas.microsoft.com/mapi/proptag/0x3A1C001F")
Summary
The custom Excel VBA function retrieves specific attributes from the Outlook address book, including local contacts and Exchange users, by querying based on a name or alias. To use the function, you need to enable the Microsoft Outlook Object Library in VBA, create a new module for the code, and then apply the function in workbook formulas to fetch various properties like job title, company name, or department.