How to Display the File Last Updated Date in Power BI Using Excel VBA or Power Query
In this example, I will demonstrate two methods to display the last updated date for an Excel file in Power BI. Both methods use an Excel file as the data source, although the second method can work with any file type. To clarify, these methods don’t rely on the Power BI timestamp for when the report was published or last refreshed. Instead, they either retrieve the last save time from Excel or use the Date modified
timestamp from the file system.
Instructions
Method 1: Excel VBA
The first method uses a custom VBA function in Excel to retrieve the Last Save Time
property.
Step 1: Create a User-Defined Function (UDF) to Retrieve Last Save Time
- From the
Developer
ribbon in Excel, clickVisual Basic
. - The
Microsoft Visual Basic for Applications
window will open. - From the
Insert
menu, clickModule
to add a new module (namedModule1
in this example). - Open
Module1
and add the following code to create the custom function that retrieves the last save timestamp:
Option Explicit
Function Last_Saved_Timestamp() As Date
On Error GoTo errorHandler
With Application
.ScreenUpdating = False
.EnableEvents = False
End With
Last_Saved_Timestamp = ThisWorkbook.BuiltinDocumentProperties("Last Save Time")
errorHandler:
With Application
.ScreenUpdating = True
.EnableEvents = True
End With
If Err.Number <> 0 Then
MsgBox Err.Description
End If
End Function
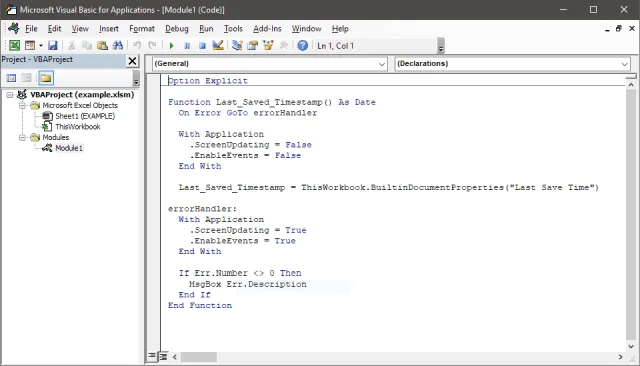
Step 2: Force Full Recalculation Before Save
- From Microsoft Visual Basic for Applications, open
ThisWorkbook
. - Add the following code. This code forces a full recalculation of the workbook and updates the timestamp before saving the file.
- Close the
Microsoft Visual Basic for Applications
window.
Option Explicit
Private Sub Workbook_BeforeSave(ByVal SaveAsUI As Boolean, Cancel As Boolean)
On Error GoTo errorHandler
With Application
.ScreenUpdating = False
.EnableEvents = False
End With
Application.CalculateFull
errorHandler:
With Application
.ScreenUpdating = True
.EnableEvents = True
End With
If Err.Number <> 0 Then
MsgBox Err.Description
End If
End Sub
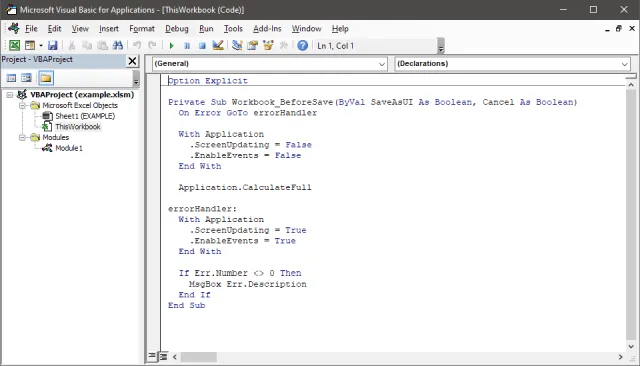
Step 3: Retrieve Last Save Time
- From Excel, create a new worksheet (named
EXAMPLE
in this case). - In cell
A1
, enterLAST SAVED DATE
. - In cell
A2
, use the custom VBA function by entering:
=Last_Saved_Timestamp()
- The timestamp will appear as a serial number.
- Save and close the Excel file.
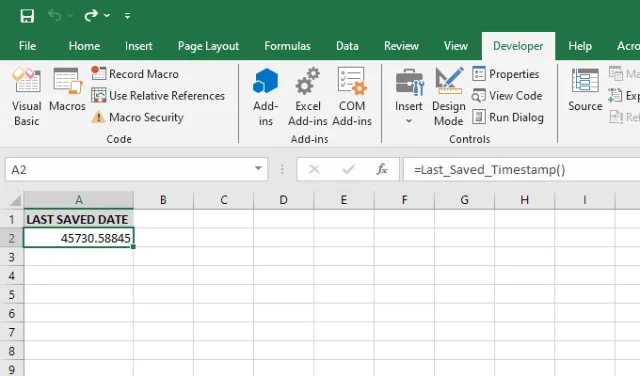
Step 4: Load Data into Power BI
- From the
Home
ribbon in Power BI Desktop, clickGet data
and selectExcel workbook
. - Select the Excel file you created earlier and click the
Open
button. - In the
Navigator
window, checkEXAMPLE
and click theLoad
button. - The
EXAMPLE
table and theLAST SAVED DATE
field will be available in theData
pane. - If
LAST SAVED DATE
is summarized, turn off summarization. In theData
pane, clickLAST SAVED DATE
, then from theColumn tools
ribbon, selectDon’t summarize
from theSummarization
drop-down. - In the
Data type
drop-down, selectDate
. If prompted with aData type change
warning, click theYes
button. - In the
Format
drop-down, choose your desired date format.

Step 5: Display Data Last Updated Date Using a Card Visualization
- Switch to
Report
view, add aCard
visualization, and dragLAST SAVED DATE
fromEXAMPLE
into theFields
section. - If it defaults to
Earliest
, change it toLatest
. This step may not be necessary if there’s only one entry in the table, but I recommend changing it toLatest
just in case. - In the
Format
section, turn off theCategory label
and adjust the font size and other attributes as needed.
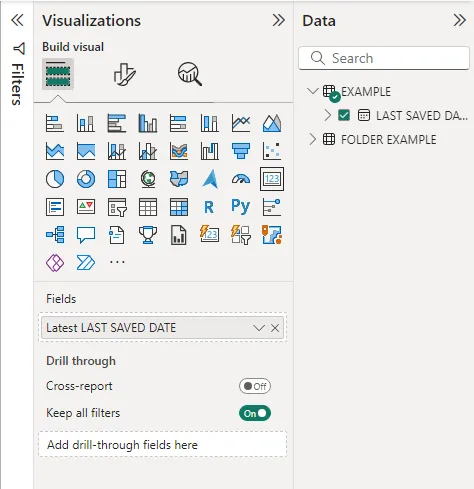
Method 2: Power Query File Properties
The second method uses Power Query to retrieve the Date modified
timestamp from the file system properties. This method works with any file type, not just Excel.
Step 1: Get Data
- From the
Home
ribbon in Power BI Desktop, clickGet data
and selectMore...
. - In the
Get Data
dialog box, chooseFile
and thenFolder
, and click theConnect
button. - In the
Folder
dialog box, enter or browse to the parent folder of the data file. Click theOK
button. - Another dialog box will appear, listing the files in the selected folder. Click the
Transform Data
button. - The
Power Query
window will open. The first step in the query is labeledSource
, which returns the file list. Verify that the list is correct. - Filter the
Name
column so that only the file used by the Power BI report appears. - Select the
Date modified
column. From theHome
ribbon, selectRemove Columns
andRemove Other Columns
. - Rename the
Date modified
column toLAST SAVED DATE
to maintain consistency with the first method. - In the
Query Settings
pane underProperties
, rename the query to something descriptive, likeFOLDER EXAMPLE
. This name will appear in theData
pane in Power BI. - From the
Home
ribbon, clickClose & Apply
.
Here is the final query code after applying all filters and transformations:
let
Source = Folder.Files(
"C:\test"
),
#"Filtered Rows" = Table.SelectRows(Source, each ([Name] = "example.xlsm")),
#"Removed Other Columns" = Table.SelectColumns(#"Filtered Rows", {"Date modified"}),
#"Renamed Columns" = Table.RenameColumns(
#"Removed Other Columns",
{{"Date modified", "LAST SAVED DATE"}}
)
in
#"Renamed Columns"
NOTE: In this demonstration, the file is located at
C:\test\example.xlsm
. Adjust the query as necessary.
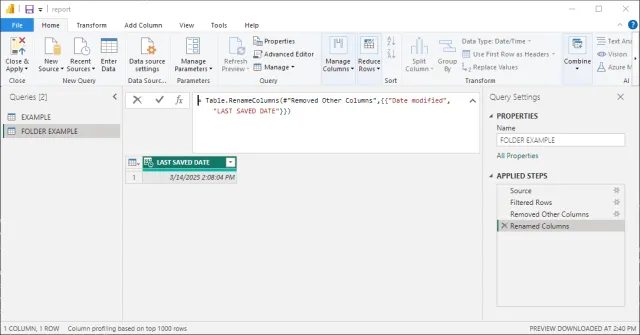
Step 2: Load Data into Power BI
- Returning to Power BI, the
FOLDER EXAMPLE
table and theLAST SAVED DATE
field will be available in theData
pane. - If
LAST SAVED DATE
is summarized, turn off summarization. In theData
pane, clickLAST SAVED DATE
, then from theColumn tools
ribbon, selectDon’t summarize
from theSummarization
drop-down. - In the
Data type
drop-down, selectDate
. If prompted with aData type change
warning, click theYes
button. - In the
Format
drop-down, choose your desired date format.
Step 3: Display Data Last Updated Date Using a Card Visualization
- Switch to
Report
view, add anotherCard
visualization, and dragLAST SAVED DATE
fromFOLDER EXAMPLE
into theFields
section. - If it defaults to
Earliest
, change it toLatest
. This step may not be necessary if there’s only one entry in the table, but I recommend changing it toLatest
just in case. - In the
Format
section, turn off theCategory label
and adjust the font size and other attributes as needed.
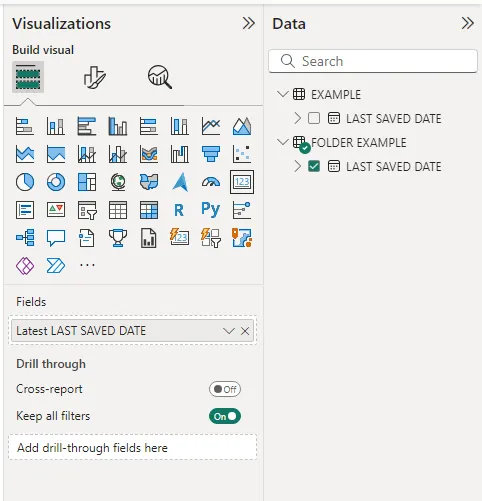
Result
Both methods will produce the same result, as shown in the screenshot below. The report displays the last saved date from either the Excel VBA method or the file system’s Date modified
timestamp. When the Power BI report is refreshed, the card visualizations will update with the latest date.
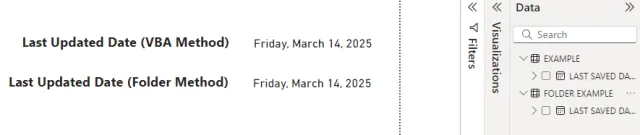
Summary
Both methods allow you to display the last updated date of your data in Power BI, either through Excel VBA or Power Query. The Excel VBA method retrieves the last save time from within Excel, while the Power Query method uses the file system’s Date modified
timestamp. Choose the method that best suits your data source and reporting needs.